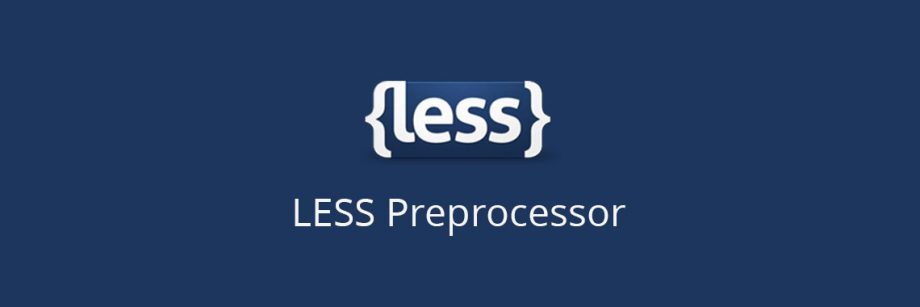
LESS – ยุคใหม่ของ CSS – 2
จากความเดิมตอนที่แล้ว LESS – ยุคใหม่ของ CSS ไม่นึกว่าจะยาวขนาดนี้
เลยยกมาเป็น Part 2 ซะเลย มาเริ่มกันเลยดีกว่าครับ
Get Started #2
4. Nested rules การเขียนเป็น ชั้นๆ
Featured นี้สามารถทำให้เราเขียน CSS ได้ง่ายขึ้นมากครับ (แนะนำๆ)
// In LESS you can nest your rulesets. // This is a very important feature because: // -You don't have to write out repeating long // selectors // -It helps to structurize your code #content { width: 500px; a { color: white; // You can use the '&'-selector to // apply a nested selector to the // parent selector: &:hover { color: blue; } &.selected { color: yellow } } }
#content { width: 500px; } #content a { color: white; } #content a:hover { color: blue; } #content a.selected { color: #ffff00; }
5. Operations ก็เป็นการคำนวณคณิตศาสตร์ทั่วๆไปครับ
// In LESS you can operate on any number, // color or variable. // Basic operations @base: 200px; @height: @base * 2; @min-width: @base + 100; @max-width: (@min-width - 50) * 2; .basic_operations { height: @height; min-width: @min-width; max-width: @max-width; } // Operating on colors: @base-color: #444; .color_operations { color: @base-color / 4; background-color: @base-color + #111; }
// Output .basic_operations { height: 400px; min-width: 300px; max-width: 500px; } .color_operations { color: #111111; background-color: #555555; }
6. Color functions เป็น Function ที่ LESS มีมาให้นะครับเกี่ยวกับพวกสีต่างๆ
นอกจากหมวดสีแล้วยังมี อย่างอื่นด้วยนะครับ ลองเข้าไปชมที่ Official LESS documentation ได้เลยครับ
// Using the functions to set a property // works as expected: @base: #f04615; .class { color: saturate(@base, 5%); background-color: lighten(spin(@base, 8), 25%); }
// Output .class { color: #f6430f; background-color: #f8b38d; }
7. Namespaces
// In LESS you can use namespaces. This can // help you to organize your code, and it // offers you encapsulation. // We declare the '.button'-mixin in the // '#myNamespace' namespace. #myNamespace { .button() { display: block; border: 1px solid black; background-color: grey; &:hover { background-color: white; } } } // Using the '.button'-mixin: #header a { color: red; #myNamespace > .button(); }
// Output #header a { color: red; display: block; border: 1px solid black; background-color: grey; } #header a:hover { background-color: white; }
ทำไมต้องมี Namespace ด้วยสร้าง mixin แบบ ธรรมดาไม่ได้หรอ ?
ประโยชน์ของ Namespace คือทำให้มันเปลี่ยนกลุ่มก้อนเดียวกันครับ
จะได้เข้าใจได้ง่ายว่ามันอยู่ในหมวดอะไร เช่น animation ของ css มันจะมีหลายแบบ
เวลาเราเรียกใช้ก็จะเป็น #animation > .animation(...)
อะไรแบบนี้ครับ มันทำให้เป็นหมวดหมู่
// Animation #animation { .animation(...) { -webkit-animation: @arguments; -moz-animation: @arguments; -ms-animation: @arguments; -o-animation: @arguments; animation: @arguments; } .animation-name(...) { -webkit-animation-name: @arguments; -moz-animation-name: @arguments; -ms-animation-name: @arguments; -o-animation-name: @arguments; animation-name: @arguments; } .animation-delay(...) { -webkit-animation-delay: @arguments; -moz-animation-delay: @arguments; -ms-animation-delay: @arguments; -o-animation-delay: @arguments; animation-delay: @arguments; } }
8. Scope
// Scope in LESS is very similar to that of // programming languages. Variables and mixins // are first looked up locally, and if they // aren't found, the compiler will look in the // parent scope, and so on. @var: red; #page { @var: white; #header { color: @var; // white } } #footer { color: @var; // red }
// Output #page #header { color: #ffffff; } #footer { color: #ff0000; }
9. Comments
จะมี 2 แบบครับ ถ้า comment แบบ //
จะไม่มีมาที่ Output CSS ครับ
แต่ถ้า comment แบบ /*...*/
ก็จะมีมาให้เห็นใน Output CSS
// CSS-style comments are preserved by LESS, // LESS-style comments are 'silent', they // don't show up in the compiled CSS output. /* Hello, I'm a CSS-style comment */ // Hi, I'm a silent comment, I won't show up // in your CSS .class { color: black }
/* Hello, I'm a CSS-style comment */ .class { color: #000000; }
10. Importing
จะเป็นการ import ไฟล์อื่นเข้ามาเพื่อทำให้เราเรียกใช้ mixin หรือ ตัวแปรที่เราได้กำหนดไว้ในไฟล์อื่นได้ครับ
// You can import .less files, and all the // variables and mixins in them will be made // available to the main file. The .less // extension is optional, so both of these are // valid: // @import "lib.less"; // @import "lib"; // If you want to import a CSS file, and don't // want LESS to process it, just use the .css // extension: // @import "lib.css"; // And to show that it works we import the // winLESS.org LESS file: @import "/style/less/main";
11. String interpolation ตัวแปร String นั่นเองครับ
// Variables can be embeded inside strings in // a similar way to ruby or PHP, with the // @{name} construct: @base-url: "http://assets.fnord.com"; .container { background-image: url("@{base-url}/images/bg.png"); }
.container { background-image: url("http://assets.fnord.com/images/bg.png"); }
12. Escaping
จะช่วยในการเล่นกับ string ครับ ในตัวอย่างจะเป็นการเล่นกับ `String interpolation`
// Sometimes you might need to output a CSS // value which is either not valid CSS syntax, // or uses propriatery syntax which LESS // doesn't recognize. // To output such value, we place it inside a // string prefixed with ~, for example: @border-preset: "1px solid #f1f1f1"; .class { filter: ~"progid:DXImageTransform.Microsoft.AlphaImageLoader(src='image.png')"; color: ~"@eee"; margin: ~"0 20px"; border: ~"@{border-preset}"; }
// Output .class { filter: progid:DXImageTransform.Microsoft.AlphaImageLoader(src='image.png'); color: @eee; margin: 0 20px; border: 1px solid #f1f1f1; }
13. Javascript สามารถใช้งานร่วมกับ Javascript ได้
// You can also use the previously mentioned interpolation: @str: "howdy"; // @str becomes 'HOWDY' @str-before: `"happy".toUpperCase()`; // becomes 'HOWDY' @str-after: ~`'@{str}'.toUpperCase()`; // Here we access part of the document @height: `document.body.clientHeight`; .class { height: @height; } .class:before { content: @str-before; } .class:after { content: @str-after; }
// Output .class { height: 1332; } .class:before { content: "HAPPY"; } .class:after { content: "HOWDY"; }
จบแล้วครับ คงได้เห็นประโยชน์ของ CSS Preprocessor ตัวนี้กันแล้ว อย่าลืมไปทดลองเล่นกันนะครับ